Football API Integration
A beginners guide on how to get started with Highlightly's Football API integration into your project to create your very own soccer platform.
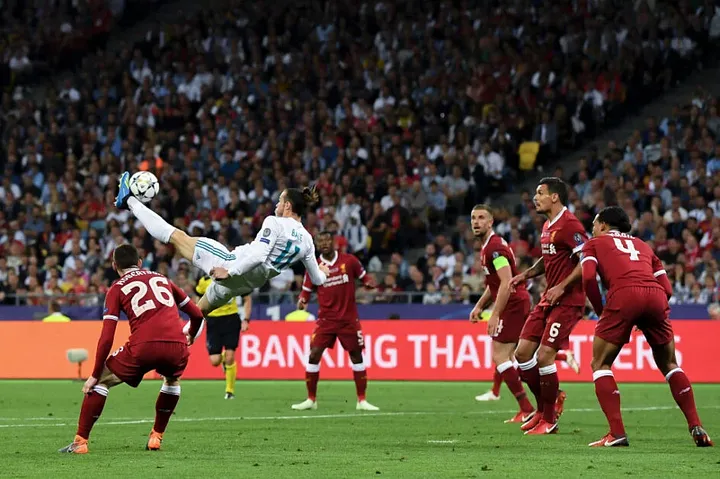
In 2025 there there are many API providers which offer a wide spectrum of sport data. Unfortunately, there are still two common issues that feed providers face:
- lack of real time odds,
- lack of real time highlights.
On the market, there are already different production ready solutions that provide betting or highlight related data but never both. Fortunately, Highlightly's Football API integrates all of the above. Pre-match and live odds coupled with multiple prediction models to assist you as well as real time live highlights, game recaps and even post match interviews.
Prerequisites for API integration
As a developer you can start using the API either through RapidAPI or Highlightly.
Accounts do not sync across platforms. Before subscribing make sure to check what the differences are.
Once you are successfully logged in, you can opt for the BASIC tier of the API which is free. However, there are certain restrictions when retrieving data such as odds or highlights. PRO plan and above removes any restrictions.
The API token you receive should be securely stored on your server. Make sure not to expose it to your clients.
Fetching football highlights data
Given the current state of the programming language popularity, we will show you how you can fetch football highlights data with Javascript.
There are a couple of main routes which are used for data fetching:
-
/countries
and/countries/:{countryCode}
-
/teams
and/teams/:{teamId}
-
/leagues
and/leagues/:{leagueId}
-
/matches
and/matches/:{matchId}
-
/highlights
and/highlights/:{highlightId}
Country, team and league routes more or less serve a utility purpose.
Lets create a simple function that will trigger a HTTP request to fetch highlights data:
const axios = require("axios");
const fetchFootballHighlightsData = async (queryParams) => {
const options = {
method: "GET",
url: "https://football-highlights-api.p.rapidapi.com/highlights",
params: queryParams,
headers: {
"X-RapidAPI-Key": "<API TOKEN>",
"X-RapidAPI-Host": "football-highlights-api.p.rapidapi.com",
},
};
try {
const response = await axios.request(options);
console.log(response.data);
} catch (error) {
console.error(error);
}
};
Make sure to add your own “X-RapidAPI-Key” which is received by subscribing to any tier of the API either on RapidAPI or Highlightly. With this, we can fetch the data however we want. E.g.:
await fetchFootballHighlightsData({
date: "2025-03-16",
timezone: "Etc/UTC"
});
The above function call would result in the following data:
{
"data": [
{
"id": 325790,
"type": "VERIFIED",
"imgUrl": "https://i.ytimg.com/vi/ak1fiHwWiTI/hqdefault.jpg",
"title": "HIGHLIGHTS | Slavia 🅱️ - Vyškov 0:0 | 19. kolo ChN Ligy",
"description": null,
"url": "https://www.youtube.com/watch?v=ak1fiHwWiTI",
"embedUrl": "https://www.youtube.com/embed/ak1fiHwWiTI",
"channel": "SK Slavia Praha",
"source": "youtube",
"match": {
"id": 1031583835,
"round": "Regular Season - 19",
"date": "2025-03-16T10:00:00.000Z",
"country": {
"code": "CZ",
"name": "Czech Republic",
"logo": "https://highlightly.net/soccer/images/countries/CZ.svg"
},
"awayTeam": {
"id": 6280313,
"logo": "https://highlightly.net/soccer/images/teams/6280313.png",
"name": "Vyškov"
},
"homeTeam": {
"id": 7334702,
"logo": "https://highlightly.net/soccer/images/teams/7334702.png",
"name": "Slavia Praha II"
},
"league": {
"id": 295230,
"logo": "https://highlightly.net/soccer/images/leagues/295230.png",
"name": "FNL",
"season": 2024
}
}
},
{
"id": 325784,
"type": "VERIFIED",
"imgUrl": "https://i.ytimg.com/vi/4mh7Zt9Cs_s/hqdefault.jpg",
"title": "Radu si oppone in tutti i modi al Napoli | Top Moment | Venezia-Napoli | Serie A Enilive 2024/25",
"description": null,
"url": "https://www.youtube.com/watch?v=4mh7Zt9Cs_s",
"embedUrl": "https://www.youtube.com/embed/4mh7Zt9Cs_s",
"channel": "Serie A",
"source": "youtube",
"match": {
"id": 1041526068,
"round": "Regular Season - 29",
"date": "2025-03-16T11:30:00.000Z",
"country": {
"code": "IT",
"name": "Italy",
"logo": "https://highlightly.net/soccer/images/countries/IT.svg"
},
"awayTeam": {
"id": 419476,
"logo": "https://highlightly.net/soccer/images/teams/419476.png",
"name": "Napoli"
},
"homeTeam": {
"id": 440751,
"logo": "https://highlightly.net/soccer/images/teams/440751.png",
"name": "Venezia"
},
"league": {
"id": 115669,
"logo": "https://highlightly.net/soccer/images/leagues/115669.png",
"name": "Serie A",
"season": 2024
}
}
},
...
],
"plan": {
"tier": "PRO",
"message": "All data available with current plan."
},
"pagination": {
"totalCount": 698,
"offset": 0,
"limit": 40
}
}
The API supports a whole variety of query parameters not just the date and timezone as presented in the above example (you can use query fields like leagueName, leagueId, season, countryCode, countryName, etc.).
Since it would take a long time to go through all examples, we suggest that you visit the RapidAPI or Highlightly endpoint testing pages and play with the API a bit.
Wrapping up
Highlights are one of the core functionalities that attract users to sport platforms. By using Highlightly's Football API you can get real time updates in just a few lines of code. But that is not all what we have to offer. Check out the documentation for in-depth explanation on how to retrieve pre-match/live odds, detailed match statistics and even match prediction.